Qt5 Signal Slot Lambda
Posted By admin On 31/07/22Signals and slots are loosely coupled: A class which emits a signal neither knows nor cares which slots receive the signal. Qt's signals and slots mechanism ensures that if you connect a signal to a slot, the slot will be called with the signal's parameters at the right time. Signals and slots can take any number of arguments of any type. Connect QML signal to C11 lambda slot(Qt 5) (2) Instead of creating lambda functions on the fly to deal with different signals, you may want to consider using a QSignalMapper to intercept the signals and send them to a statically-defined slot with an argument dependent on the source. Just like a classic signal-slot connection, if the context object thread is not the same as the code emitting the signal, Qt will use a queued connection. Alternative to QMetaObject::invokeMethod. QObject has also non-static versions of connect so you can connect signal to slot as follows: receiver-connect(sender,signal,slot); Of course, receiver must be an object of some class derived of QObject. Things go pretty much well until the introduction of lambda expression in recent Qt versions. Now you can connect a signal to a lambda.
- Qt5 Signal Slot Lambda Bot
- Qt5 Signal Slot Lambda Alpha
- Qt5 Signal Slot Lambda Pi
- Qt5 Signal Slot Lambda Python
Introduction
Remarks
Official documentation on this topic can be found here.
A Small Example
Signals and slots are used for communication between objects. The signals and slots mechanism is a central feature of Qt and probably the part that differs most from the features provided by other frameworks.
The minimal example requires a class with one signal, one slot and one connection:
counter.h
The main
sets a new value. We can check how the slot is called, printing the value.
Finally, our project file:
Connecting overloaded signals/slots
While being better in many regards, the new connection syntax in Qt5 has one big weakness: Connecting overloaded signals and slots. In order to let the compiler resolve the overloads we need to use static_cast
s to member function pointers, or (starting in Qt 5.7) qOverload
and friends:
Multi window signal slot connection
A simple multiwindow example using signals and slots.
There is a MainWindow class that controls the Main Window view. A second window controlled by Website class.
The two classes are connected so that when you click a button on the Website window something happens in the MainWindow (a text label is changed).
I made a simple example that is also on GitHub:
mainwindow.h
mainwindow.cpp
website.h
website.cpp
Project composition:
Consider the Uis to be composed:
- Main Window: a label called 'text' and a button called 'openButton'
- Website Window: a button called 'changeButton'
So the keypoints are the connections between signals and slots and the management of windows pointers or references.
The new Qt5 connection syntax
The conventional connect
syntax that uses SIGNAL
and SLOT
macros works entirely at runtime, which has two drawbacks: it has some runtime overhead (resulting also in binary size overhead), and there's no compile-time correctness checking. The new syntax addresses both issues. Before checking the syntax in an example, we'd better know what happens in particular.
Let's say we are building a house and we want to connect the cables. This is exactly what connect function does. Signals and slots are the ones needing this connection. The point is if you do one connection, you need to be careful about the further overlaping connections. Whenever you connect a signal to a slot, you are trying to tell the compiler that whenever the signal was emitted, simply invoke the slot function. This is what exactly happens.
Here's a sample main.cpp:
Hint: the old syntax (SIGNAL
/SLOT
macros) requires that the Qt metacompiler (MOC) is run for any class that has either slots or signals. From the coding standpoint that means that such classes need to have the Q_OBJECT
macro (which indicates the necessity to run MOC on this class).
The new syntax, on the other hand, still requires MOC for signals to work, but not for slots. If a class only has slots and no signals, it need not have the Q_OBJECT
macro and hence may not invoke the MOC, which not only reduces the final binary size but also reduces compilation time (no MOC call and no subsequent compiler call for the generated *_moc.cpp
file).
I have a mainWindow class that calls a function mainWIndow::ShowDialogBox()
when double clicked on the QTabBar. The dialog box shows up, but it isn't connecting the buttons. I have the connect calls in ShowDialogBox. It gives me a red underline on connect saying
This is my code
I have added the signals and slot in mainWindow.h as
I have spend hours on this but no luck. I am new to Qt.
Line:
should be:
Because the third parameter has to be a memory address(pointer).
How can I anchor widgets in Qt Creator?
python,qt,layout,qt-designer
You need to add a layout to the top widget. You need to right-click the most outside widget (Dialog), select 'Lay out' and select appropriate layout (grid layout will do fine). This will ensure that direct children of the Dialog will react to its size changes. To prevent stretching ListWidget...

Attempting to read stdout from spawned QProcess
qt,signals-slots,qprocess
Issue is in statement QObject::connect(console_backend, SIGNAL(console_backend ->readyReadStandardOutput()), this, SLOT(this->receiveConsoleBackendOutput())); It should be QObject::connect(console_backend, SIGNAL(readyReadStandardOutput()), this, SLOT(receiveConsoleBackendOutput())); ...
How to implement Recent Files action with Qt designer?
c++,qt,qt-designer
When I tried to implement the Recent Files I come up with a solution like this: I create the menù items on startup and set them non visible: separatorAct = ui.menu_File->addSeparator(); for (int i = 0; i < MAXRECENTFILE; ++i) { RecentProjects[i] = new QAction(this); RecentProjects[i]->setVisible(false); connect(RecentProjects[i], SIGNAL(triggered()), this, SLOT(OpenRecentFile()));...
Cannot connect (null)::selectionChanged to QTableView
c++,qt,qt-creator,signals-slots,qtableview
The signal slot connection has failed since table->selectionModel() has returned null. If you set the model for your table before making signal slot connection, table->selectionModel() will return a valid model, making the signal slot connection successful....
Overriding/Reimplementing Slots in PySide
python,qt,override,pyside,signals-slots
You cannot override QLineEdit.copy or QLineEdit.paste in such a way that they will be called internally by Qt. In general, you can only usefully override or reimplement Qt functions that are defined as being virtual. The Qt Docs will always specify whether this is the case, and for QLineEdit, there...
qt 'emit' signal not working properly
c++,qt,signals-slots,emit
Remove the multiple instances of dosecalibration, or make sure to connect each one of those, if you really need multiple instances.
How to connect signal to boost::asio::io_service when posting work on different thread?
c++,multithreading,boost,boost-asio,signals-slots
In light of new information, the problem is with your boost::bind. You are trying to call a member function without an object to call it on: you are trying to call ProcessData but you haven't told the bind on which object you wish to call it on. You need to...
QTableView / QTableWidget: Stretch Last Column using Qt Designer
pyqt,pyqt4,qt-designer,pyqt5
In the Qt Designer, Select the QTableWidget / QTableView and navigate to the Property Editor. Here, scroll down to the 'Header section' and enable horizontalHeaderStretchLastSection. ...
PyQt: Wrapping Dialog from QDesigner and Connect pushbutton
python,pyqt,signals-slots,qpushbutton,pyuic
The problem in the original code is in this section: if True: qApp = QtGui.QApplication(sys.argv) Dialog = QtGui.QDialog() u = Testdialog() u.setupUi(Dialog) Dialog.exec_() sys.exit(qApp.exec_()) What you want instead is something like this: if __name__ '__main__': app = QtGui.QApplication(sys.argv) u = Testdialog() u.show() sys.exit(app.exec_()) The reason why the original code...
C++: Thread Safety in a Signal/Slot Library
c++,multithreading,thread-safety,signals-slots
I suspect there is no clearly good answer, but clarity will come from documenting the guarantees you wish to make about concurrent access to an Emitter object. One level of guarantee, which to me is what is implied by a promise of thread safety, is that: Concurrent operations on the...
QtDesigner for Raspberry Pi
qt4,raspberry-pi,qt-creator,qt-designer
To run qt-creator on Rpi itself. You can install it by 'sudo apt-get install qt-creator' It will install qt-creator and qt4-base modules on rpi. After installing, you can run 'qt-creator' on terminal to get started with design. You will be using drag and drop for UI design and c++ as...
Can't emit signal in QML custom Item
qt,qml,signals-slots
Functions (which signals are) are first class objects in JS, so it is not an error to refer to them without parentheses. But you need them in order to execute the function (i.e. emit the signal). So just change this line: onClicked: baseButton.clicked() ...
PyQt4 signals and slots - QToolButton
python,qt,pyqt4,signals-slots
I'm not sure why you're doing the following, but that's the issue: def showSettings(self): dialog = QtGui.QDialog() dialog.ui = SettingsDialog() dialog.ui.setupUi(dialog) dialog.exec_() SettingsDialog itself is a proper QDialog. You don't need to instantiate another QDialog. Right now, you're creating an empty QDialog and then populate it with the same ui...
Qt5 Signal Slot Lambda Bot
How to get value of QtComboBox?
python,qt,pyqt,qt-designer
Where you say 'city' you should say 'self.city' so that city is attached to the object. Then later you can get its text as 'self.city.currentText()'.
Drag n Drop Button and Drop-down menu PyQt/Qt designer
qt,python-2.7,pyqt,pyqt4,qt-designer
In order to add code to a UI generated with QtDesigner, you must generate a .py file using pyuic: pyuic myform.ui -o ui_myform.py This ui_myform.py file, contains generated code that you should not edit, so later you can change your .ui file with QtDesigner, re-run pyuic, and get ui_myform.py updated...
Dynamic mapping of all QT signals of one object to one slot
c++,qt,signals-slots
have a look at const char * QMetaMethod::signature() const then you should be able to use it like QObject::connect(object, method->signature(), this, SLOT(signalFired())); you might need to add '2' before the method->signature() call because SIGNAL(a) makro is defined SIGNAL(a) '2'#a as mentioned Is it possible to see definition of Q_SIGNALS, Q_SLOT,...
PyQt5 - Signals&Slots - How to enable a button through content change of lineEdit?
button,signals-slots,pyqt5,qlineedit
Looks like you want the textChanged signal, since that sends the current text: self.lineEdit_SelectedDirectory.textChanged.connect( lambda text: self.pushButton_CreateFileList.setEnabled(bool(text))) ...
How to dock horizontally Qt widgets?
c++,qt,qt-creator,qt-designer
In Qt, widget geometry can be automatically managed by layouts. A widget by itself won't fill the parent. You need to set a layout on the parent widget, and add the widget to the parent. There are numerous tutorials on that. The particular layout that would apply to your situation...
Qt:signal slot pass by const reference
c++,qt,pass-by-reference,signals-slots,pass-by-value
When you pass an argument by reference, a copy would be sent in a queued connection. Indeed the arguments are always copied when you have a queued connection. So here there would be no trouble regarding the life time of images since it will be copied instead of passed by...
Cross-thread signal slot, how to send char *
c++,multithreading,qt,signals-slots
The problem you have is completely unrelated to Qt, signals or multiple threads. The char* you're creating isn't null-terminated, so you cannot use it with functions (or operators) that expect char*s to be C strings - they rely on the null terminator. What you're seeing on your console is the...
Qt: How to remove the default main toolbar from the mainwindow?
c++,qt,qt-creator,qt-designer,qtgui
You need to remove the main toolbar from either QtDesigner as can be seen below or from the code manually: Then, you will need to rerun qmake for the changes to take effect as the ui_mainwindow.h header file needs to be regenerated from the updated mainwindow.ui description file. ...
Signals and Slots in Qt4
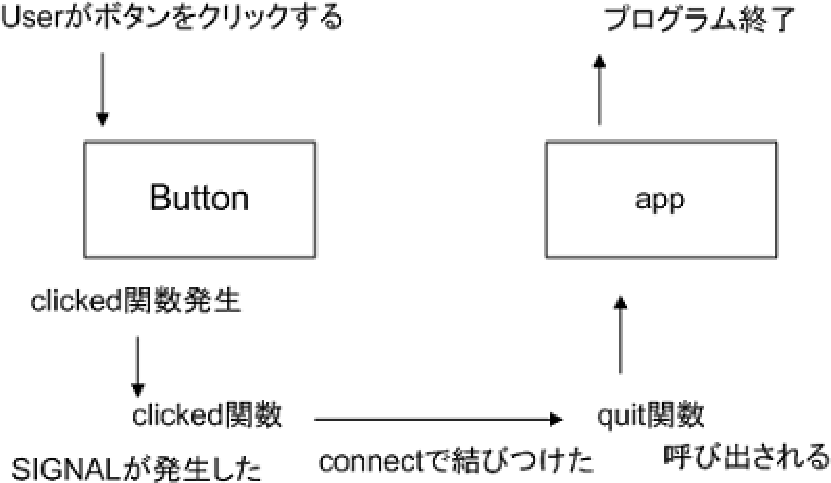
c++,qt,qt4,signals-slots,qt4.7
Basically you need to connect your signal and slot connect(ui->button1, SIGNAL(clicked()), this, SLOT(yourSlot())); and in this link there is good example about signals and slot: signals and slots in qt....
Syntax sugar for signal slot
c++,c++11,signals-slots
Is it possible in modern C++ to implement such functionality in, for example, C# way? [...] No, that's not possible in C++. The syntax for taking the address of a member function requires qualifying the function name with the class name (i.e. &MyClassName::myMethodName). If you don't want to specify...

Event loops and signal-slot processing when using multithreading in Qt
c++,multithreading,qt,event-loop,signals-slots
All results you got are perfectly correct. I'll try to explain how this works. An event loop is an internal loop in Qt code that processes system and user events. Event loop of main thread is started when you call a.exec(). Event loop of another thread is started by default...
C++ Signal QML Slot on registered type
c++,qml,signals-slots,qt5.4
I hadn't realized that I had connected to the wrong instance of MsgController in my code. One instance was created by the C++ code, but I really wanted to call the slot on the instance created by the Qml Engine. Once I connected to the correct instance, the code above...
Qt app receives HUP signal unexpectedly when forked to background
qt,signals,fork,daemon,signals-slots
When daemonizing (forking), the parent process issues a HUP signal upon exit. For some reason on Red Hat this signal doesn't hit child process until much later. On Ubuntu the signal hits the child quickly (or perhaps Ubuntu holds the signal for the child). Solution is to confirm parent process...
Qt Designer: Unable to get scroll area to work properly
qt,scroll,qt-designer
Apply a layout to the Window QWidget (which layout, is irrelevant) and place the scroll area inside. (answering the last phase of my question so I don't have to delete it, after gradually figuring out how to make it work)...
How to manage QSplitter in Qt Designer
c++,qt,qt-creator,qt-designer,qsplitter
You can simply create splitter containing items in Qt Designer : First place your widgets on your dialog or widget in designer (They should not be in a layout) Select the widgets that you want to be in a splitter (By holding CTL and clicking on them) Right click on...
PyQt QTreeview not displaying first column of QAbstractItemModel when built with Qt Designer
python,qt,pyqt,qt-designer
Change: self.treeView.setRootIsDecorated(False) To: self.treeView.setRootIsDecorated(True) ...
Pyside: Multiple QProcess output to TextEdit
python,pyside,signals-slots,qprocess
Connect the signal using a lambda so that the relevant process is passed to the slot: p.readyReadStandardOutput.connect( lambda process=p: self.write_process_output(process)) def write_process_output(self, process): self.viewer.text_edit.append(process.readAllStandardOutput()) ...
Iterating all items inside QListView using python
python,pyqt4,qt-designer
Use the model to iterate over the items: model = self.listView.model() for index in range(model.rowCount()): item = model.item(index) if item.isCheckable() and item.checkState() QtCore.Qt.Unchecked: item.setCheckState(QtCore.Qt.Checked) ...
QTabWidget Content Not Expanding
c++,xml,user-interface,qt5,qt-designer
To set the layout on a tab, you first need to select its parent tab-widget. You can do this either by selecting it by name in the Object Inspector (i.e. by clicking on xMarketTabWidget in your example), or by simply clicking on an appropriate tab in its tab-bar. The parent...
Getting rid of QtTabWidget margins
qt,qt-designer
Sounds like you might want documentMode: ...
PyQt5: one signal comes instead of two as per documentation
python,pyqt,python-3.3,signals-slots,pyqt5
You only connected to one of the two signal overloads. Since you also didn't specify which overload you wanted, a default will be selected - which in this case will be valueChanged(int). To explicitly select both overloads, you would need to do: self.spb.valueChanged[int].connect(self.onValueChanged) self.spb.valueChanged[str].connect(self.onValueChanged) ... def onValueChanged(self, x): print('QSpinBox: value...
Qt: Connect inside constructor - Will slot be invoked before object is initialized?
c++,qt,event-handling,signals-slots
You don't have to worry about such scenarios in most cases, because events are delivered in the same thread. There's no 'hidden multithreading' going on you have to care about. If you don't explicitly call a function in the constructor of A that causes events to be processed, you're safe...
Calling a C++ function from Qt (slot does not work)
c++,qt,signals-slots
Just register a Qt slot within your header file. class Log { ... Q_SLOTS: void sendMessage (); } and implement the slot in the implementation, e.g. by: Log::sendMessage() { send_message(); } Do not forget to change connect statement to just the sendMessage() function rather than your send_message function....
How to close Pyqt5 program from QML?
qt,python-3.x,qml,signals-slots,pyqt5
There are a few syntax errors in the python script, but ignoring those, the code can be made to work like this: def main(argv): app = DestinyManager(sys.argv) engine = QtQml.QQmlEngine(app) engine.quit.connect(app.quit) ... Which is to say, you simply need to connect the qml quit signal to an appropriate slot in...
qt slots and signalls autoconnecting
qt,qt5,signals-slots,qt5.2
I fixed it by accident while writing this question... My model was missing a name. When I added kontrahentModel->setObjectName('kontrahentModel'); All worked like a charm... BUT - 'there is no rose without a thorn'. When assigning a parent for the model, an old problem returns - described here: QSqlQueryModel with a...
How to resolve 2 sequential calls on Qt slot and perform action only once
c++,qt,lambda,signals-slots,qt-signals
You can apply next pattern to your code: class MyClass : public QObject { private slots: void updateRequest(); private: QTimer *_timer; CodeEditor *_editor; }; MyClass::MyClass() { // Init members // ... _timer->setSingleShot( true ); _timer->setInterval( 0 ); connect( _editor, &CodeEditor:: cursorPositionChanged, _timer, &QTimer::start); connect( _editor, &CodeEditor:: selectionChanged, _timer, &QTimer::start); connect(...
How to declare New-Signal-Slot syntax in Qt 5 as a parameter to function
c++,qt,qt5,signals-slots
You should create template: template<typename Func> void waitForSignal(const typename QtPrivate::FunctionPointer<Func>::Object *sender, Func signal) { QEventLoop loop; connect(sender, signal, &loop, &QEventLoop::quit); loop.exec(); } Usage: waitForSignal(button, &QPushButton::clicked); ...
How often are objects copied when passing across PyQt signal/slot connections?
python,pyqt,signals-slots,pyqt5
As suggested by @ekhumoro, I did try, and surprisingly I get a different result to what the test conducted in C++ reveals. Basically, my test shows that: objects are not copied at all, even when passed across thread boundaries using QueuedConnection Consider the following test code: class Object2(QObject): def __init__(self):...
PyQt: ListWidget.insertItem not shown
python,pyqt,signals-slots,qt-designer,qlistwidget
There is only one button in your dialog, and so it will become the auto-default. This means that whenever you press enter in the dialog, the button will receive a press event, even if it doesn't currently have the keyboard focus. So the item does get added to the list-widget...
How to execute a function in Qt when a variable changes its value in the QML?
c++,qt,signals,signals-slots
Using your example regarding ints, it would be done like this: class foo : public QObject { Q_OBJECT Q_PROPERTY( int value READ getValue WRITE setValue NOTIFY valueChanged ) public: explicit foo( QObject* parent = nullptr ) : QObject{ parent }, i_{ 0 } {} virtual ~foo() {} int getValue() const...
Disconnecting signals fails
c++,qt,signals-slots
I've checked how QObject::disconnect is implemented and I don't see how this is supposed to work if you only specify receiver. QMetaObjectPrivate::disconnect will return immediately with false when sender is not specified. This means that second part of QObject::disconnect will no set res to true. The only other place you...
connecting signals and slots with different relations
c++,qt,connect,signals-slots
You need to create new slot for that purpose. But in C++ 11 and Qt 5 style you can use labmdas! It is very comfortable for such short functions. In your case: connect(ui->horizontalSlider, &QSlider::sliderMoved, this, [this](int x) { this->ui->progressBar->setValue(x / 2); }); ...
Qt/C++ how to wait a slot when signal emitted
c++,multithreading,qt5,signals-slots
My first thought is that having either thread block until an operation in the other thread completes is a poor design -- it partially defeats the purpose of having multiple threads, which is to allow multiple operations to run in parallel. It's also liable to result in deadlocks if you're...
QT Designer how to assign 2 hotkeys to a QPushButton (python3)
Qt5 Signal Slot Lambda Alpha
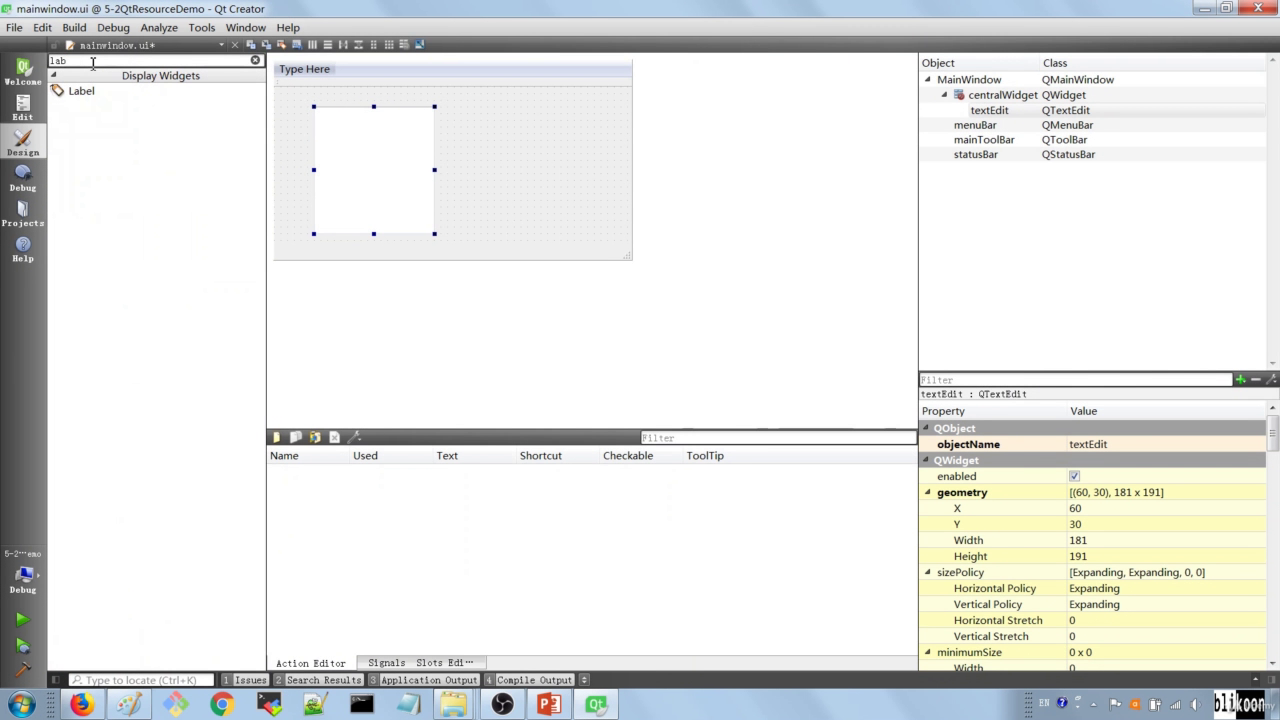
python-3.x,keyboard-shortcuts,qt-designer,pyqt5,qpushbutton
There are several ways to do this. Probably the simplest is to use QShortcut: QShortcut(Qt.Key_Enter, self.Begin, self.handleBegin) QShortcut(Qt.Key_Return, self.Begin, self.handleBegin) To get the button animation behaviour, try this instead: QShortcut(Qt.Key_Enter, self.Begin, self.Begin.animateClick) QShortcut(Qt.Key_Return, self.Begin, self.Begin.animateClick) ...
PyQt: slot is called many times
python,pyqt,signals-slots
Qt5 Signal Slot Lambda Pi
The reason why the output is being printed three times, is because of the way you named the signal handlers. The connect slots by name feature will automatically connect signal handlers that are named using the following format: on_[object name]_[signal name] Since the clicked signal has two overloads, and you...
Qt - Connect slot with argument using lambda
Qt5 Signal Slot Lambda Python
python,qt,lambda,pyqt,signals-slots
The issue is python's scoping rules & closures. You need to capture the group: def connections(self): for group in self.widgets: self.widgets[group].clicked.connect(lambda g=group: self.openMenu(g)) def openMenu(self,group): print group ...